My love language is
if (future === now) {
hostAndDeliverData({
mechanism: "decentralized"
});
} else {
getLeftBehind(you);
}
AIOZ Web3 Service is a smarter, more efficient and more affordable way to store and deliver your data.
The cloud storage generation is evolving to a diversified network.

< secure >
Advanced encryption is applied to secure your data; only you own full access to your storage.

< scalable >
W3S adapts continuously to your growing storage needs.

< affordable >
No hidden costs, just transparent pricing for everyone from startups to enterprise.
50,000
Active edge Nodes
96%
less bandwidth cost
15MB/s
average download speed
Built for your framework
AIOZ W3S integrates with your existing codebase.
1package main
2
3import (
4 "context"
5 "log"
6 "os"
7 "strings"
8
9 "github.com/aws/aws-sdk-go-v2/aws"
10 "github.com/aws/aws-sdk-go-v2/config"
11 "github.com/aws/aws-sdk-go-v2/service/s3"
12)
13
14func main() {
15 resolver := aws.EndpointResolverWithOptionsFunc(func(service, region string, options ...interface{}) (aws.Endpoint, error) {
16 return aws.Endpoint{
17 URL: "https://s3.w3s.storage/",
18 SigningRegion: "us-east-1",
19 }, nil
20 })
21
22 credentials := aws.CredentialsProviderFunc(func(ctx context.Context) (aws.Credentials, error) {
23 return aws.Credentials{
24 AccessKeyID: "YOUR_ACCESS_KEY_ID",
25 SecretAccessKey: "YOUR_SECRET_ACCESS_KEY",
26 }, nil
27 })
28
29 // Load config
30 cfg, err := config.LoadDefaultConfig(context.TODO(),
31 config.WithCredentialsProvider(credentials),
32 config.WithEndpointResolverWithOptions(resolver),
33 )
34 if err != nil {
35 log.Fatal(err)
36 }
37
38 // Create an Amazon S3 service client
39 s3Client := s3.NewFromConfig(cfg,
40 func(o *s3.Options) {
41 o.UsePathStyle = true
42 },
43 )
44
45 filePath := "YOUR_FILE_PATH"
46 // Get file name from filePath
47 path := strings.Split(filePath, "/")
48 fileName := path[len(path)-1]
49
50 file, openErr := os.Open(filePath)
51 if openErr != nil {
52 log.Fatal(openErr)
53 }
54 defer file.Close()
55 _, putErr := s3Client.PutObject(context.TODO(), &s3.PutObjectInput{
56 Bucket: aws.String("YOUR_BUCKET_NAME"),
57 Key: aws.String(fileName),
58 Body: file,
59 })
60
61 if putErr != nil {
62 panic(putErr)
63 }
64
65 log.Println("Successfully uploaded object")
66}
67
1import { S3Client, PutObjectCommand } from "@aws-sdk/client-s3";
2import path from "path";
3import fs from "fs";
4
5// Create an Amazon S3 service client object.
6const s3Client = new S3Client({
7 region: "us-east-1",
8 credentials: {
9 accessKeyId: "YOUR_ACCESS_KEY_ID",
10 secretAccessKey: "YOUR_SECRET_ACCESS_KEY",
11 },
12 endpoint: {
13 url: "https://s3.w3s.storage"
14 },
15 forcePathStyle: true,
16});
17
18const filePath = "YOUR_FILE_PATH";
19
20// Set the bucket parameters
21export const bucketParams = {
22 Bucket: "YOUR_BUCKET_NAME",
23 Key: path.basename(filePath),
24 Body: fs.createReadStream(filePath),
25};
26
27// Upload a file to AIOZ Web3 Storage
28export const run = async () => {
29 try {
30 const data = await s3Client.send(new PutObjectCommand(bucketParams));
31 console.log("Success", data);
32 } catch (err) {
33 console.log("Error", err);
34 }
35};
36
37run();
1import os
2import sys
3
4import boto3
5from botocore.exceptions import ClientError
6from botocore.config import Config
7
8def main():
9 s3_resource = boto3.resource('s3',
10 region_name='us-east-1',
11 # Get the credentials from the environment variables.
12 aws_access_key_id="YOUR_ACCESS_KEY_ID",
13 aws_secret_access_key="YOUR_SECRET_ACCESS_KEY",
14 endpoint_url='https://s3.w3s.storage',
15 config=Config(
16 # signature_version='s3',
17 s3={'addressing_style': 'path'}
18 )
19 )
20 # Create an Amazon S3 service resource object.
21 bucket_name = "YOUR_BUCKET_NAME"
22 file_path = "YOUR_FILE_PATH"
23
24 bucket = s3_resource.Bucket(bucket_name)
25 object_key = os.path.basename(file_path)
26 bucket = bucket.Object(object_key)
27
28 # Upload a file to AIOZ Web3 Storage
29 with open(file_path, 'rb') as data:
30 try:
31 result = bucket.put(Body=data)
32 bucket.wait_until_exists()
33 print(f"Success", result)
34 except ClientError as e:
35 print("Error", e)
36
37if __name__ == '__main__':
38 main()
1// Integration code is on its way!
1import { S3Client, PutObjectCommand } from "@aws-sdk/client-s3";
2import path from "path";
3import fs from "fs";
4
5// Create an Amazon S3 service client object.
6const s3Client = new S3Client({
7 region: "us-east-1",
8 credentials: {
9 accessKeyId: "YOUR_ACCESS_KEY_ID",
10 secretAccessKey: "YOUR_SECRET_ACCESS_KEY",
11 },
12 endpoint: {
13 url: "https://s3.w3s.storage"
14 },
15 forcePathStyle: true,
16});
17
18const filePath = "YOUR_FILE_PATH";
19
20// Set the bucket parameters
21export const bucketParams = {
22 Bucket: "YOUR_BUCKET_NAME",
23 Key: path.basename(filePath),
24 Body: fs.createReadStream(filePath),
25};
26
27// Upload a file to AIOZ Web3 Storage
28export const run = async () => {
29 try {
30 const data = await s3Client.send(new PutObjectCommand(bucketParams));
31 console.log("Success", data);
32 } catch (err) {
33 console.log("Error", err);
34 }
35};
36
37run();


Simple S3 integration
Generate a S3 credential key to integrate into your project.
Flexible pricing
WE ACCEPT PAYMENT WITH THE AIOZ TOKEN.
Pay as you go
EXPERIENCE THE POWER OF AIOZ
W3S.
Monthly
Go Web3
Pay with $AIOZ
Use The AIOZ Native Token to deliver your content.
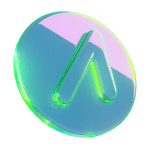
class BlockchainTechnology {
distributedBy = "p2p-nodes"
deliverySpeed = "instant"
}
Web3 Storage (W3S) uses Blockchain Technology to deliver data. Unlike Cloud providers, W3S leverages a network of distributed P2P nodes to store and deliver data.